So, can I have a private repository where composer can download my packages?
Yes, you can.
Per a request from my friends and family, I created this simple guide to install a package from a private repository that is hosted in Bitbucket.
Overview
- Create SSH keys.
- Create my package.
- Add to my repository.
- Let's do it!
Create SSH
If you already have SSH private and public keys you can skip this step.
If not see Bitbucket guide.
1. Keep in hand the public key we will use it later.
My package
1. Lets create a package called "mypackage".
composer.json
{ "name": "abelbm/mypackage", "autoload": { "psr-4": { "Abelbm\\Mypackage\\": "src" } } }
test.php
namespace Abelbm\Mypackage; class test { public function test(){ echo "test"; } }
2. Add this package in a git repository in Bitbucket with the repository name "mypackage".
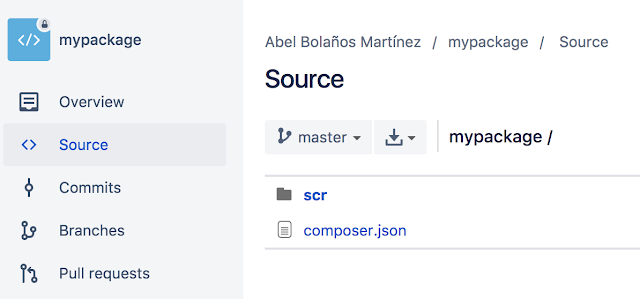
3. Push also to your repository the tag "v1.0".
git tag -a v1.0 -m "first version" git push origin v1.0
4. Check the tag in your repository.

5. Go to the repository's settings.
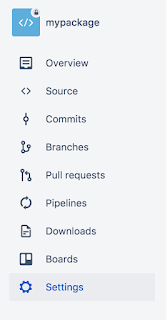
6. Navigate to the section "Access Keys".
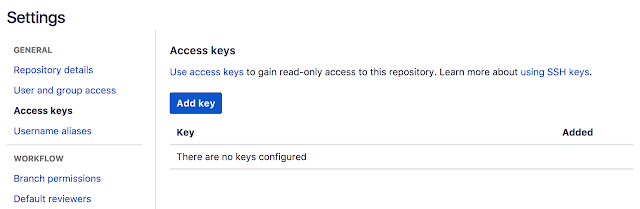
7. Add your public key.
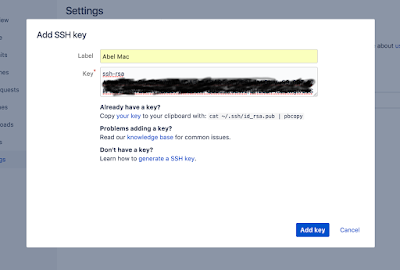
8. Done!
My project
1. Lets say we have our project called "myproject". Add a "composer.json" file.
composer.json
{ "require": { "abelbm/mypackage": "1.0" }, "repositories": [ { "type": "vcs", "url": "ENTER YOUR REPOSITORY PATH" } ] }